Tom Muck's Blog Category: SQL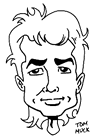
News and Views
9 posts
Showing 1
| Previous
| Next
(page 5 of 9)
Track browser resizing in your database using AJAX -- part 2
Friday, November 18, 2005 7:19:23 AM
Part 1 of this post showed the server-side code for a browser resize tracker. This part will show the client-side script. This can go on any type of page -- php, coldfusion, html, etc. The scripts consist of several functions:
- getBrowserSize() -- called in the onload and onresize event to capture the browser size and pass to the server-side page
- getSize() -- gets the size of the browser window
- passFields() -- takes an array of fields (fieldname, value, fieldname, value, etc) and a URL and passes the fields to the URL as querystring variables
- resetSizeTimer() -- creates a timer so that when the browser is resized, only one event is recorded (browser resizing typically fires the onResize event numerous times in succession.)
In addition, we set a global variable to act as a flag for the resize timer. The code is pretty straightforward, and can be placed in the head of any file:
<script>
var size_timer = false;
// Subroutine to get the size of the window
function getSize() {
var myWidth = 0, myHeight = 0;
if(typeof(window.innerWidth) == 'number') {
//Non-IE
myWidth = window.innerWidth;
myHeight = window.innerHeight;
}else if(document.documentElement &&
(document.documentElement.clientWidth || document.documentElement.clientHeight)) {
//IE 6+ in 'standards compliant mode'
myWidth = document.documentElement.clientWidth;
myHeight = document.documentElement.clientHeight;
} else if(document.body && (document.body.clientWidth || document.body.clientHeight)) {
//IE 4 compatible
myWidth = document.body.clientWidth;
myHeight = document.body.clientHeight;
}
return [myWidth, myHeight];
}
// Pass fields to server given a URL and fields in name/value pairs
function passFields(url,args) {
url += "?";
for(var i=0; i<args.length; i=i+2) {
url += args[i] + "=" + args[i+1] + "&";
}
//Set up the AJAX communication
if (window.XMLHttpRequest) {
req = new XMLHttpRequest();
} else if (window.ActiveXObject) {
req = new ActiveXObject("Microsoft.XMLHTTP");
}
try {
// Pass the URL to the server
req.open("GET", url, true);
req.send(null);
}catch(e) {
//nothing;
}
}
function resetSizeTimer() {
size_timer = false;
}
// Get the size and pass to the server
function getBrowserSize() {
if(size_timer)return;
size_timer = true;
self.setTimeout('resetSizeTimer()',1000);
var theArray = getSize();
var url = "getBrowserSize.php";
var args = new Array();
args.push("width");
args.push(theArray[0]);
args.push("height");
args.push(theArray[1]);
args.push("screenwidth");
args.push(screen.width);
args.push("screenheight");
args.push(screen.height);
args.push("pagename");
args.push(window.location);
passFields(url, args);
}
</script>
All you need to do is to add the getBrowserSize() function to the onload and onresize events of the <body> tag:
<body onload="getBrowserSize();" onresize="getBrowserSize();">
Now, when you browse the page, the server records the browser size upon load and upon resize. Typical information would look like this:
Width | Height | Screen width |
Screen height |
IP | Page name |
---|---|---|---|---|---|
856 | 788 | 1280 | 1024 | 192.168.1.2 | http://mysite.com/index.cfm |
766 | 625 | 1280 | 1024 | 192.168.1.2 | http://mysite.com/index.cfm |
948 | 751 | 1280 | 1024 | 192.168.1.2 | http://mysite.com/index.cfm |
1025 | 757 | 1280 | 1024 | 192.168.1.2 | http://mysite.com/index.cfm |
The technique is handy and can be used for any other situation where you need to pass client-side information to the server.
Cross-posted at CMXTraneous
Category tags: Dreamweaver, ColdFusion, SQL
Posted by Tom Muck
Add comment |
View comments (1) |
Permalink
|
Trackbacks (0)
|
Digg This
9 posts
Showing 1
| Previous
| Next
(page 5 of 9)
Before posting comments or trackbacks, please read the posting policy.